DATA STRUCTURE
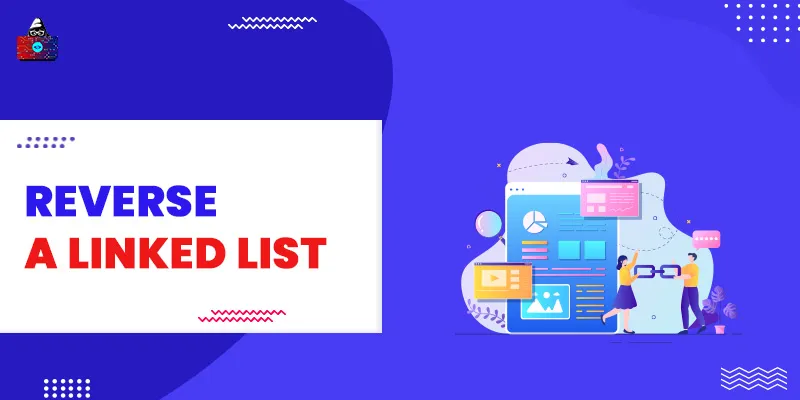
Reverse a Linked List
Linked List is one of the most important data structures in computer science due to several reasons…
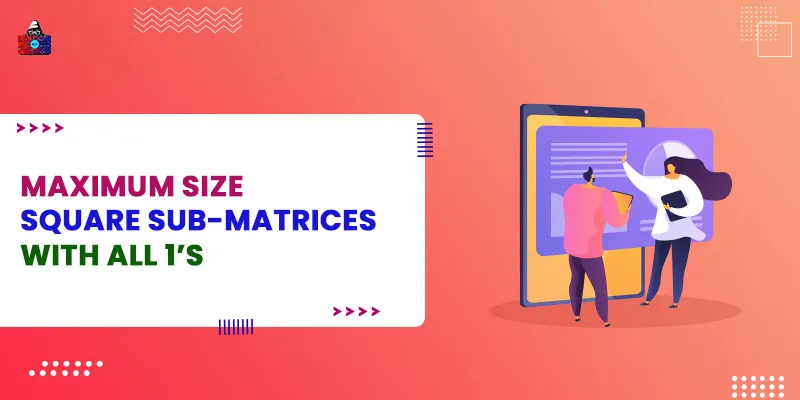
Maximum size square sub-matrices with all 1's
Problem Statement Consider a matrix with all 0s and 1s. Your task is to find the largest square …
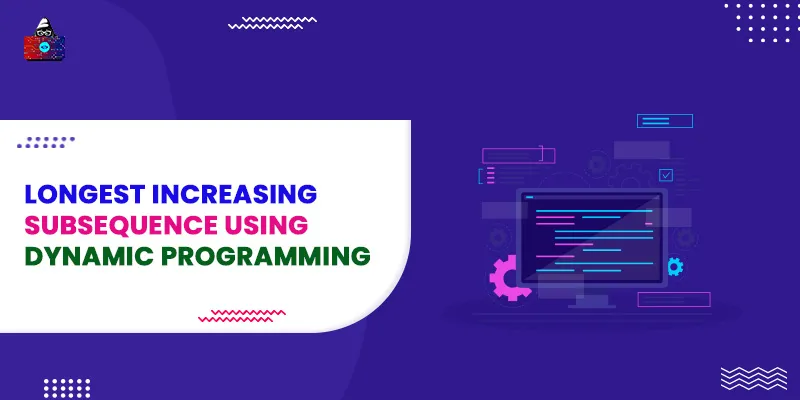
Longest Increasing Subsequence using Dynamic Programming
You are given a sequence. You have to find the length of the longest subsequence of the given seque…
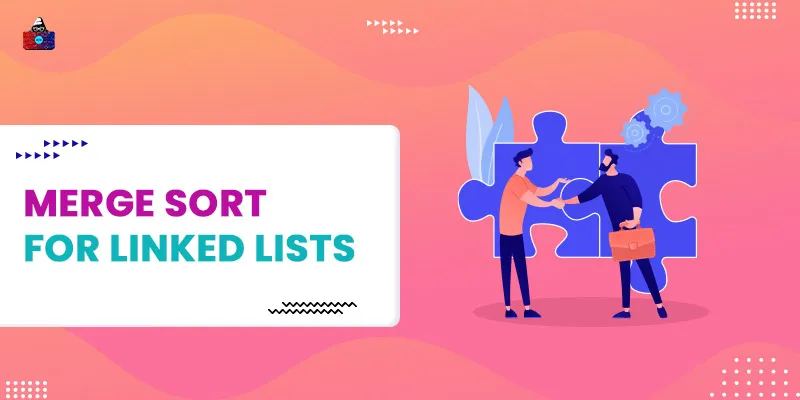
Merge Sort for Linked Lists
There are a plethora of sorting algorithms present for arrays like the bubble sort, insertion sort,…
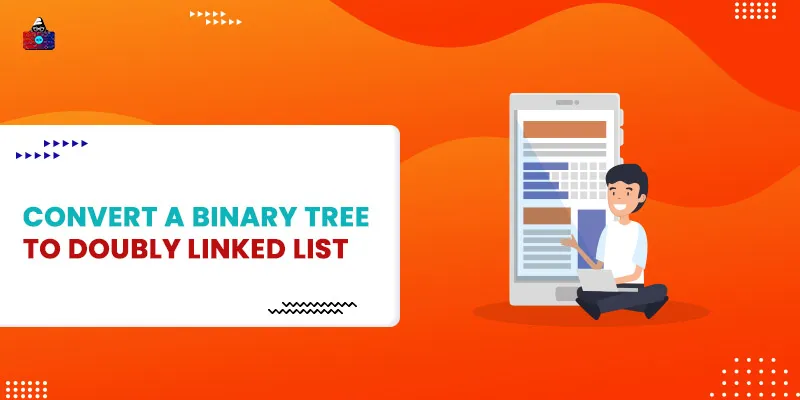
Convert a Binary Tree to Doubly Linked List
You are given a Binary Tree. Your task is to convert it into a doubly-linked list. The left and rig…
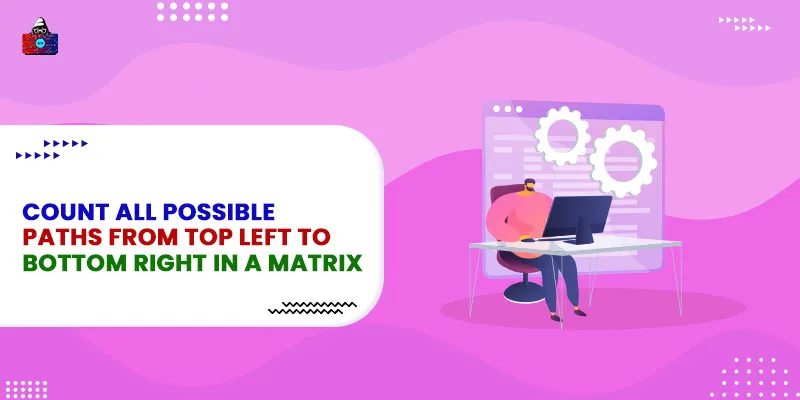
Count all Possible Paths from Top Left to Bottom Right in a Matrix
You are given a matrix of m*n dimensions. You have to calculate the total number of possible paths …
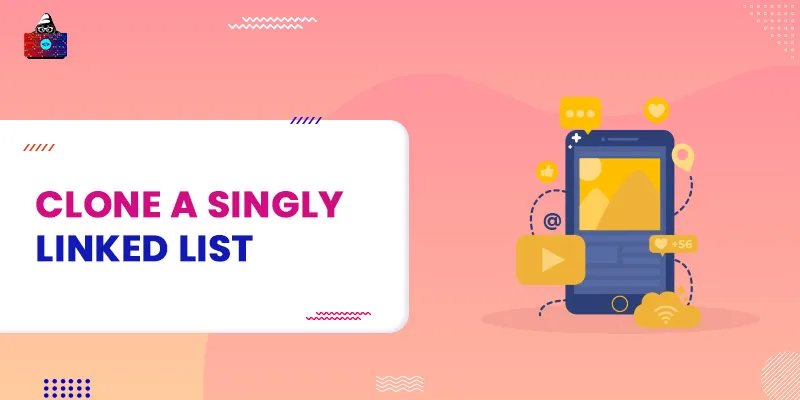
Clone a Singly Linked List
You are given a singly linked list, having n nodes in it with each node having a pointer that is po…
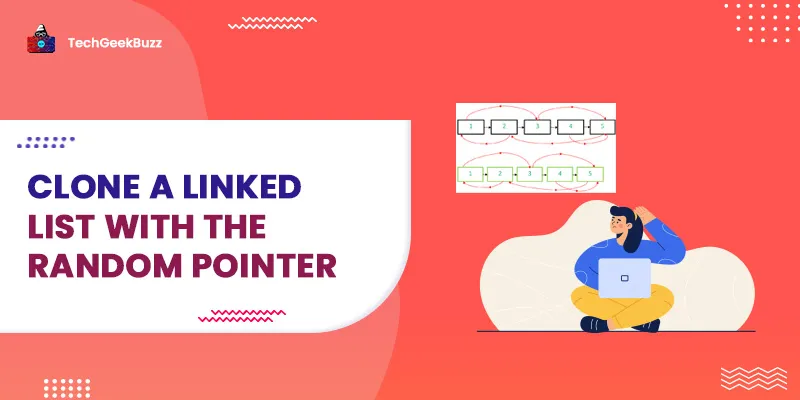
Clone a Linked list with the Random Pointer
Given a linked list, in which every node contains the next pointer. They also contain an additional…
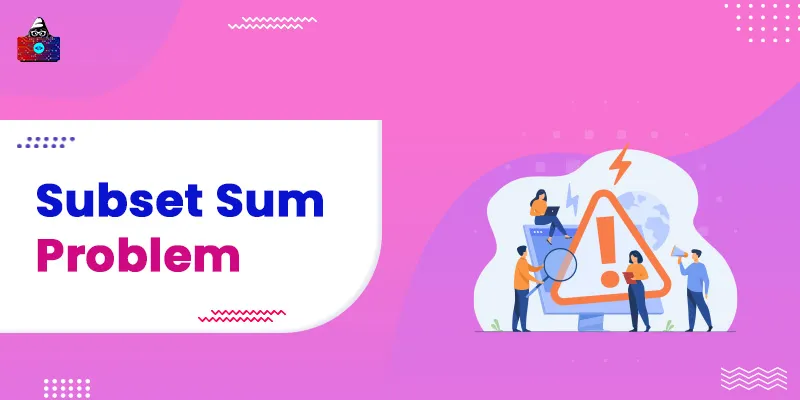
Subset Sum Problem
Subset Problem - Dynamic Problem You are given a set of positive integers (0 included) and a sum…
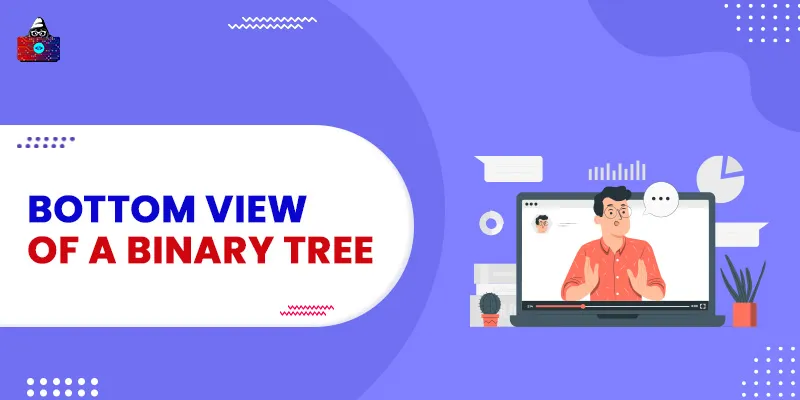
Bottom View of a Binary Tree
Bottom View of a Binary Tree You are given a Binary Tree. We will suppose the left and right chi…
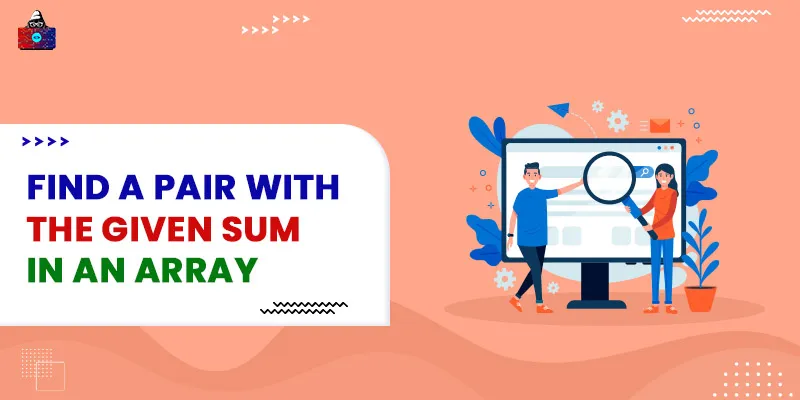
Find a Pair with the Given Sum in an Array
In this tutorial, we will implement 3 different algorithms to find all the pairs with a given sum p…
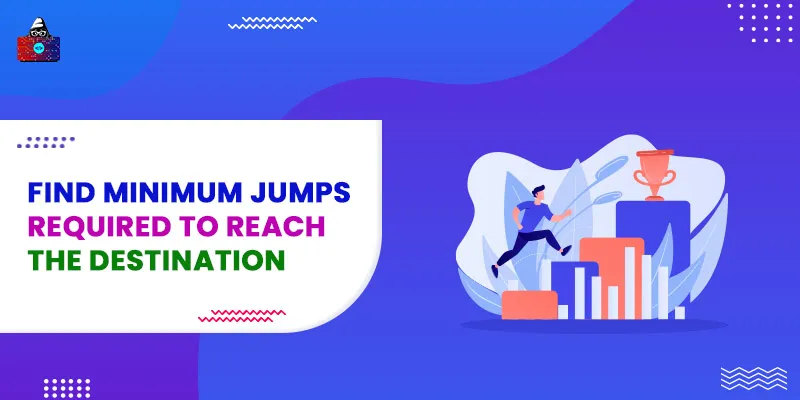
Find minimum jumps required to reach the destination
You are given a list of positive numbers. In this list, each integer denotes the maximum number of …