In the previous tutorial, we learned about JavaScript
for...loop
which syntax is similar to other programming languages for a loop. But in JavaScript, we get two more types of
for
loops for specific conditions.
- JavaScript for...in Loop
- JavaScript for...in Loop
JavaScript for..in loop
The
for..in
loop is used to iterate over the keys of the JavaScript object. In the normal
for
loop, we have the initial, condition, and increment/decrement expressions, but in
for..in
loop we have a variable and object. And the
for..in
loop iterate over the object's keys, one by one. By far we have not discussed the JavaScript object in detail, we only know that a JavaScript object is a collection of key and value pairs that we learned in the J
avaScript Data Types and Data Structure tutorial
. For now, that's all you need to know about the JavaScript objects because JavaScript is all about objects. And with the help of
for..in
loop we can iterate over the JavaScript object keys or properties.
Syntax
for (variable in object) { //for loop code block
}
Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h3> JavaScript For..in Example</h3>"); let obj ={one:"|", two:"||", three:"|||", four:"||||"} for(key in obj) { document.write(key); document.write("<br/>"); } </script> <body> </body> </html>
Output
In the output, you can see that the
for...in
loop only iterate over the keys of object
obj
. It did not iterate over the values.
JavaScript for..of loop
The
for..of
loop in JavaScript is used to iterate over the iterable objects like Array, Map, Set, arguments, string, etc. With the help of
for...of
loop we can iterate or traverse through the values of an array or every character of a string.
Syntax
for( value of iterable_object) { //for loop block }
Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h3> JavaScript For..of Example</h3>"); let arr = ["One", "Two", "Three", "Four"] for(value of arr) { document.write(value); document.write("<br/>"); } </script> <body> </body> </html>Output

arr
every value using the
for..of
loop.
Difference Between JavaScript for..in and for..of
for...in
with JavaScript object data structure and
for...of
with other iterable objects like array, set, map, string, etc.
for...in
as well as
for..of
loop, but the object can only iterate using
for..in
loop.
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h3> JavaScript For..of Example</h3>"); let arr = ["One", "Two", "Three", "Four"] document.write("<h3> Array with For..of </h3>"); for(value of arr) { document.write(value); document.write("<br/>"); } document.write("<h3> Array with For..in </h3>"); for(index in arr) { document.write(index); document.write("<br/>"); } </script> <body> </body> </html>Output
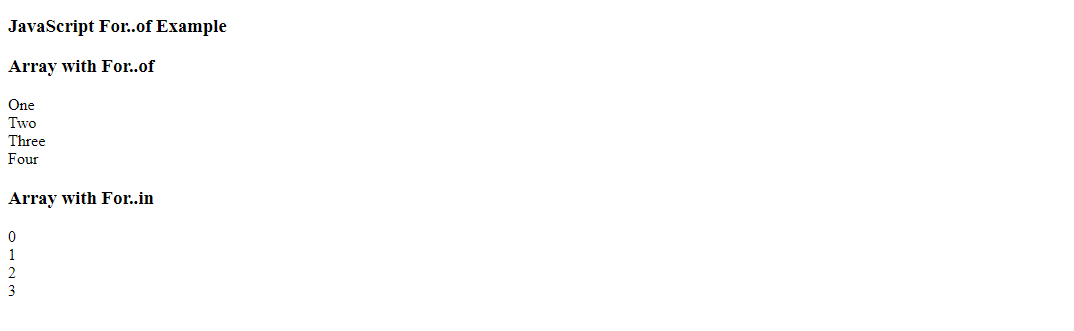
for...of
loop iterate over the
arr
values, and the
for..in
loop object iterates over its index values because an array is an object itself.
Summary
-
for...in
loop is used to iterate over the object's keys. -
for..of
loop is used to iterate over iterable objects. - With for..of and for...in loops we can eliminate the chances of the infinite loop because an object or iterable object can have limited elements.
People are also reading: